Working with labeled regions in image processing often requires not only visualizing the segments but also understanding what each color represents. The scikit-image library provides the convenient label2rgb
function to visualize labeled images by mapping labels to colors. However, one significant drawback is that label2rgb
does not automatically generate a legend. This can become a challenge when communicating results, especially in applications such as medical imaging, object detection, or geographic segmentation where clarity is essential.
This article explores practical strategies to display a legend when using label2rgb
, helping you enhance the interpretability of your visualizations. We will walk through a structured approach that includes manual legend creation using matplotlib
, and how to ensure label-to-color consistency.
Understanding label2rgb
The label2rgb
function in scikit-image converts a labeled image (usually produced by functions like skimage.measure.label
or skimage.segmentation.slic
) into an RGB image based on the unique regions. It assigns random or specified colors to each label, which can be overlaid on a grayscale background for better contrast.
However, by default, it does not expose color-label mappings, making it difficult to generate legends.
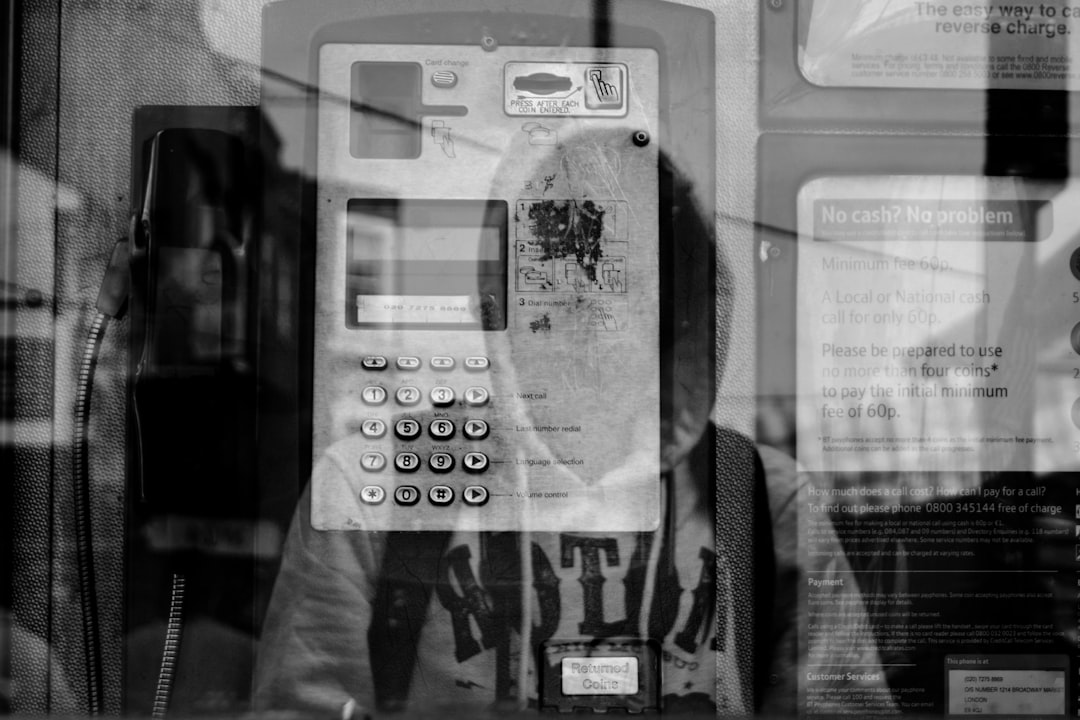
Step-by-Step Guide: Manually Creating a Legend
To create a functional and visually aligned legend, follow these steps:
-
Generate a label map
First, generate a 2D or 3D label array using segmentation methods such as watershed, connected components, or SLIC. -
Use
label2rgb
with consistent colors
To maintain consistency in visualization:- Define a custom list of colors.
- Map each label to a color manually to avoid randomness across runs.
from skimage.color import label2rgb import numpy as np custom_colors = [(1, 0, 0), (0, 1, 0), (0, 0, 1)] image_rgb = label2rgb(label_image, colors=custom_colors, bg_label=0)
-
Add a legend using
matplotlib
Manually construct a legend corresponding to your custom colors:import matplotlib.pyplot as plt from matplotlib.patches import Patch labels = ["Region A", "Region B", "Region C"] patches = [Patch(color=custom_colors[i], label=labels[i]) for i in range(len(labels))] plt.imshow(image_rgb) plt.legend(handles=patches, bbox_to_anchor=(1.05, 1), loc='upper left') plt.tight_layout() plt.show()
This method ensures that each color segment is clearly labeled and improves understandability.
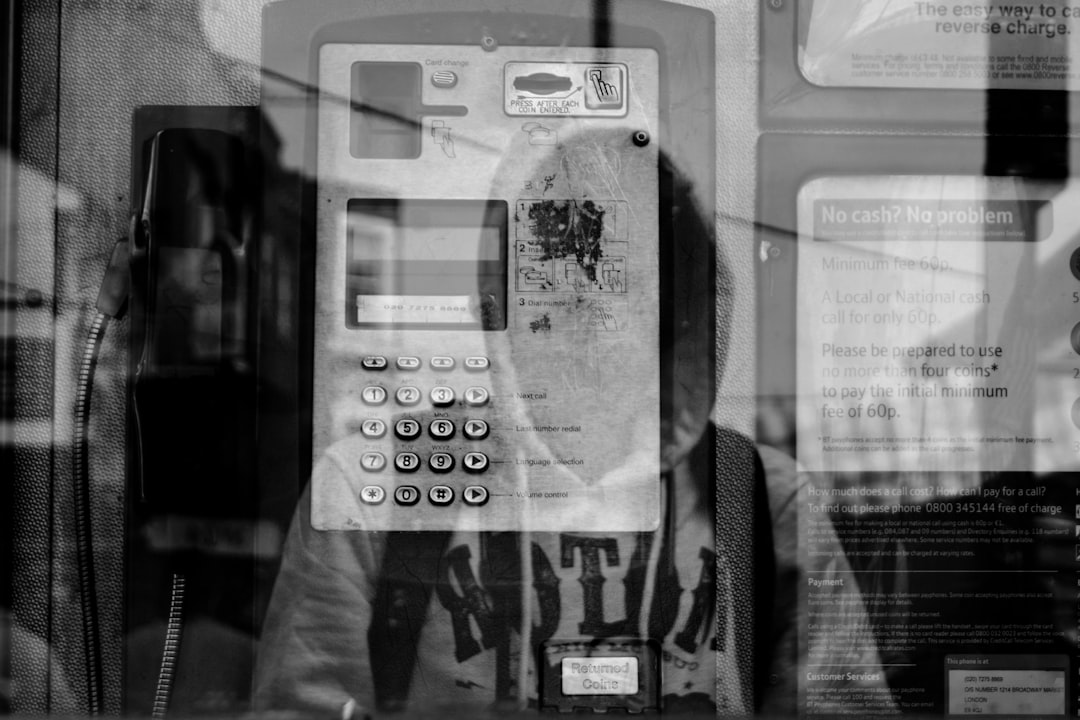
Advanced Tips and Considerations
Here are some tips for achieving the most effective visualization:
- Sort labels: Ensure that labels are sorted if they come from an automatic process where region IDs may not follow a predictable order.
-
Use colormaps: For large numbers of regions, you can use colormaps such as
matplotlib.cm.tab20
to generate a list of distinguishable colors. - Handle background labels: Make sure the background label (typically 0) has a neutral or transparent color if not assigned to a meaningful region.
Using colormaps, you can dynamically allocate a palette like this:
import matplotlib.cm as cm
num_labels = len(np.unique(label_image)) - 1 # exclude background
colormap = cm.get_cmap('tab20', num_labels)
colors = [colormap(i)[:3] for i in range(num_labels)]
image_rgb = label2rgb(label_image, colors=colors, bg_label=0)
Conclusion
Although label2rgb
does not natively support legends, with some manual steps you can create professional, informative visualizations. By combining custom color assignment with matplotlib
patches, you bridge the gap between data analysis and communicative visual storytelling.
This level of control is essential in domains where audiences rely heavily on color cues to interpret image segmentation results. Incorporating a legend not only clarifies your data but also adds a layer of credibility and completeness to your image presentations.
Remember, the clarity of your visualizations can significantly influence the impact of your work—don’t underestimate the power of a well-crafted legend.
Leave a Reply