JSON Web Tokens (JWT) provide a secure, compact, and self-contained way to transmit information between parties as a JSON object. In C#, developers often rely on JWT to implement authentication and authorization mechanisms in modern web applications, particularly when working with ASP.NET Core.
What is a JSON Web Token?
A JWT is essentially a string consisting of three parts: a header, a payload, and a signature, each encoded in Base64URL format and separated by dots:
- Header: Contains the type of the token and the signing algorithm.
- Payload: Contains the claims, which are statements about the user and custom metadata.
- Signature: Ensures the token hasn’t been tampered with, created using a secret key and the algorithm specified in the header.
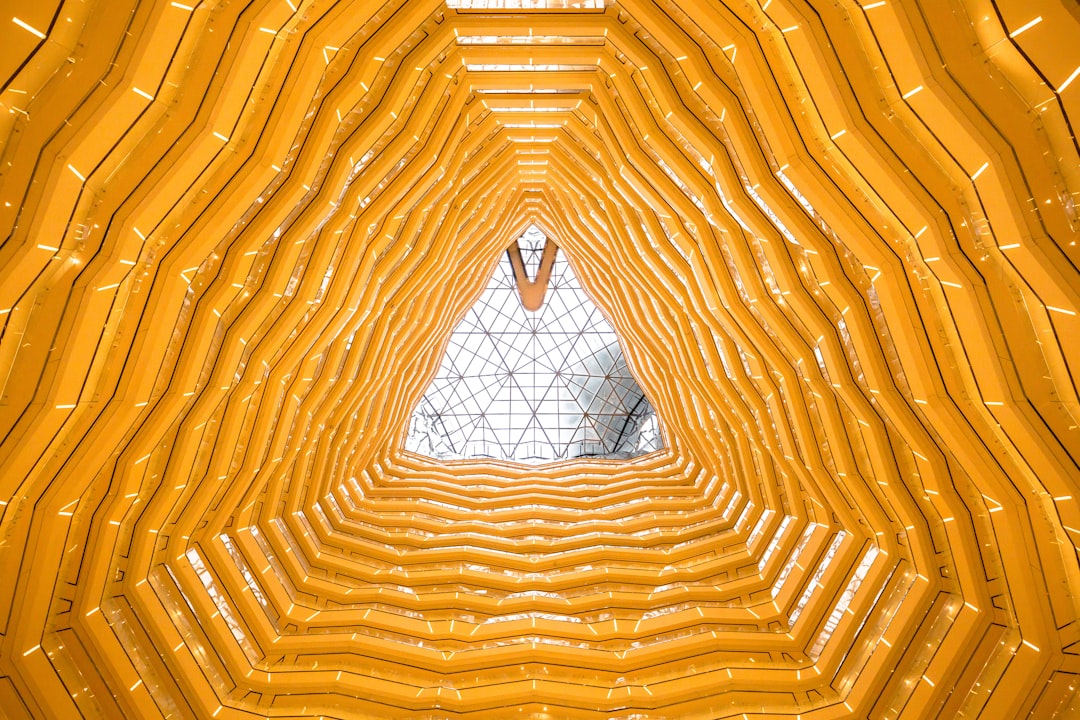
JWTs are widely used to manage session information without relying on server-side sessions. Once a user logs in, the server generates a JWT, and the client includes it in requests to protected endpoints, typically in the Authorization
header.
Generating a JWT in C#
To generate a JWT in C#, particularly in an ASP.NET Core environment, you’ll primarily be using the System.IdentityModel.Tokens.Jwt
package, along with supporting libraries:
using System;
using System.IdentityModel.Tokens.Jwt;
using System.Security.Claims;
using Microsoft.IdentityModel.Tokens;
using System.Text;
public class JwtTokenGenerator
{
public string GenerateToken(string username)
{
var securityKey = new SymmetricSecurityKey(Encoding.UTF8.GetBytes("your_secret_key_here"));
var credentials = new SigningCredentials(securityKey, SecurityAlgorithms.HmacSha256);
var claims = new[]
{
new Claim(JwtRegisteredClaimNames.Sub, username),
new Claim(JwtRegisteredClaimNames.Jti, Guid.NewGuid().ToString())
};
var token = new JwtSecurityToken(
issuer: "yourdomain.com",
audience: "yourdomain.com",
claims: claims,
expires: DateTime.Now.AddHours(1),
signingCredentials: credentials);
return new JwtSecurityTokenHandler().WriteToken(token);
}
}
This method returns a string JWT that the client can store and append to HTTP requests for authentication purposes. The secret key must be kept secure, as it’s instrumental in signing and validating tokens.
Validating a JWT in C#
Token validation ensures the integrity and origin of the token. Using middleware like JwtBearerAuthentication
in ASP.NET Core simplifies this process:
services.AddAuthentication(options =>
{
options.DefaultAuthenticateScheme = JwtBearerDefaults.AuthenticationScheme;
options.DefaultChallengeScheme = JwtBearerDefaults.AuthenticationScheme;
})
.AddJwtBearer(options =>
{
options.TokenValidationParameters = new TokenValidationParameters
{
ValidateIssuer = true,
ValidateAudience = true,
ValidateLifetime = true,
ValidateIssuerSigningKey = true,
ValidIssuer = "yourdomain.com",
ValidAudience = "yourdomain.com",
IssuerSigningKey = new SymmetricSecurityKey(Encoding.UTF8.GetBytes("your_secret_key_here"))
};
});
This configuration is added to the Startup.cs
file and instructs the application on how to authenticate and authorize incoming requests using JWTs.
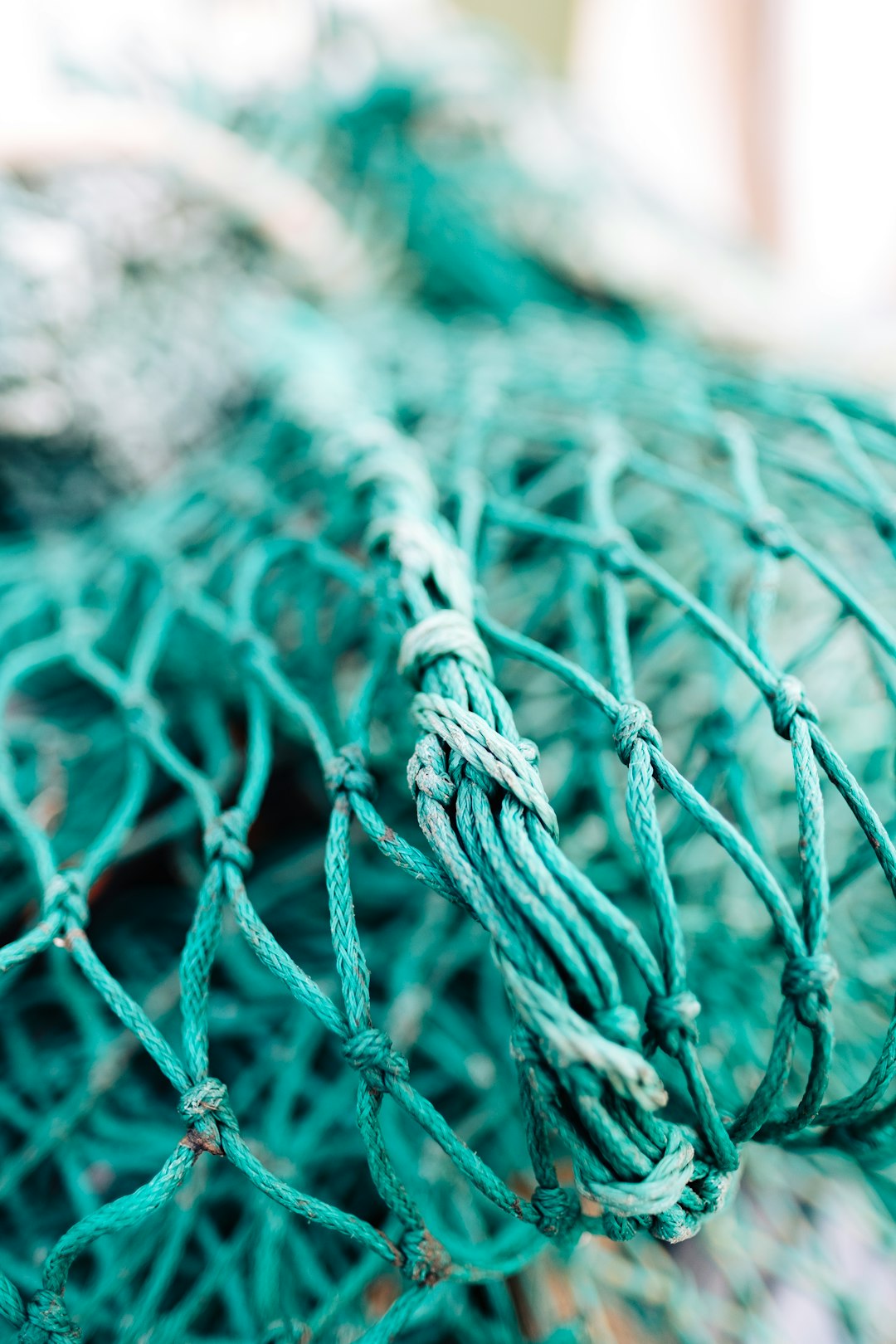
Advantages of Using JWTs in C# Applications
Developers choose JWTs for several compelling reasons:
- Stateless Authentication: No server-side session storage needed, which improves scaling.
- Cross-Language Support: JWT is a standardized format supported across different platforms and languages.
- Embedded Claims: Custom claims can be added easily to suit application-specific needs.
- Secure Transmission: With proper encryption and HTTPS, transmitted data is safe.
JWT has become a cornerstone in building RESTful APIs, especially where microservices and distributed systems are involved.
FAQ: JSON Web Tokens Using C#
-
Q: What library do I need to handle JWT in C#?
A: You needSystem.IdentityModel.Tokens.Jwt
and related packages such asMicrosoft.AspNetCore.Authentication.JwtBearer
for working within ASP.NET Core. -
Q: Are JWTs secure?
A: JWTs can be secure when used correctly—employ HTTPS, algorithm like HMAC-SHA256, and properly manage secret keys. -
Q: Can JWTs be modified by the user?
A: While users can technically decode and alter the non-signature parts, any change invalidates the signature, making the token unusable during validation. -
Q: How long should a JWT last?
A: Token expiration depends on security needs. Common practice is between 15 minutes to several hours, with refresh tokens for extending sessions.
Using JWT with C# empowers developers to build secure and efficient APIs. As long as best practices are followed, it offers a robust pathway for stateless authentication.
Leave a Reply