Cracking a C++ interview can be a bit nerve-wracking. But hey, you’ve got this! If you’re an experienced C++ developer, you know the basics. Now, it’s time to dive deeper into questions that test your knowledge and experience. So, grab a coffee, relax, and let’s go through the top 15 C++ interview questions that might pop up in your next big interview.
1. What is RAII in C++?
RAII stands for Resource Acquisition Is Initialization. It’s a design principle where resource allocation is tied to object lifetime. So when an object is destroyed, its resources are released. This makes memory and resource management safe and automatic.
2. What are smart pointers and how are they different from raw pointers?
Smart pointers like unique_ptr, shared_ptr, and weak_ptr help manage memory by automatically deleting objects when they’re no longer needed. They help prevent memory leaks, unlike raw pointers.
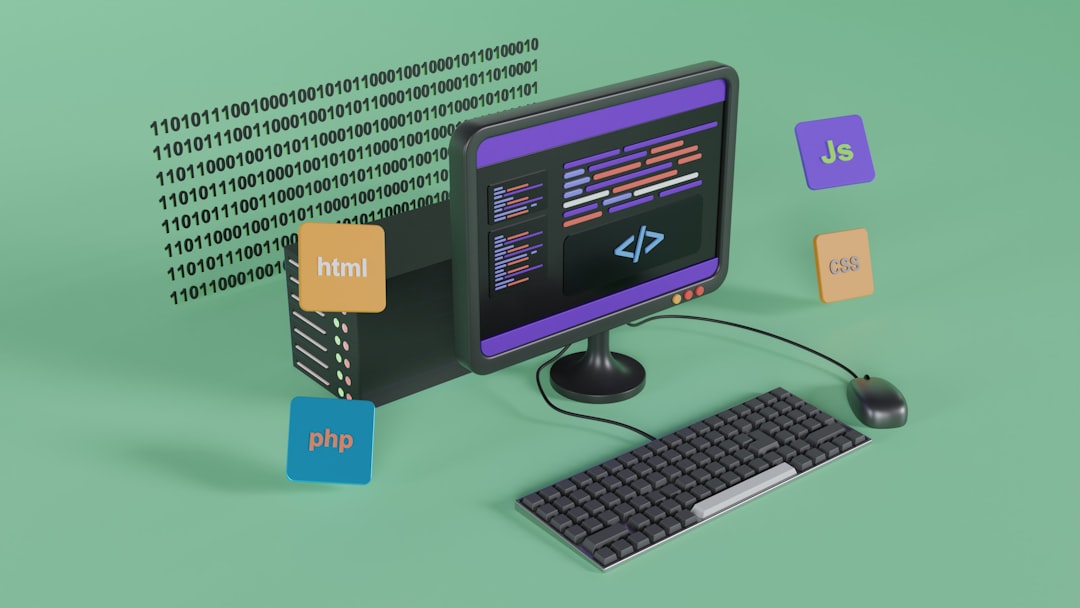
3. What is the Rule of Five?
The Rule of Five says that if you implement any one of these — destructor, copy constructor, copy assignment, move constructor, move assignment — you probably need to implement all five. It’s about managing resources cleanly when using custom classes.
4. What’s the difference between deep copy and shallow copy?
Shallow copy copies pointers, so two objects point to the same data. Deep copy copies the actual content. Deep copies are safer when you don’t want shared access to the internal data.
5. What are lambda functions?
Lambdas are anonymous functions you can define right inside your code. They’re super useful when passing small functions as arguments.
auto sum = [](int a, int b) { return a + b; };
6. Explain the difference between stack and heap allocation.
- Stack: Fast, automatically managed memory. Size is limited.
- Heap: Dynamically allocated using new and delete.
Use the stack when possible; prefer the heap when you need more control.
7. What’s the use of const
keyword?
const
means a variable or function can’t change the data it refers to. It makes your code safer and easier to understand.
8. What is inheritance and how does it work with polymorphism?
Inheritance lets one class use methods and properties of another. With polymorphism, a base class pointer can call derived class methods using virtual functions.
9. Explain the difference between compile-time and run-time polymorphism.
- Compile-time (Static): Function overloading, templates.
- Run-time (Dynamic): Virtual functions.
10. What are virtual destructors?
If a base class has virtual functions, it should also have a virtual destructor. Why? Because when you delete a derived class object through a base class pointer, it ensures the destructor of the derived class runs properly.
11. Can you explain move semantics?
Move semantics allow transferring resources from one object to another, instead of copying. This improves performance — especially in resource-heavy applications.
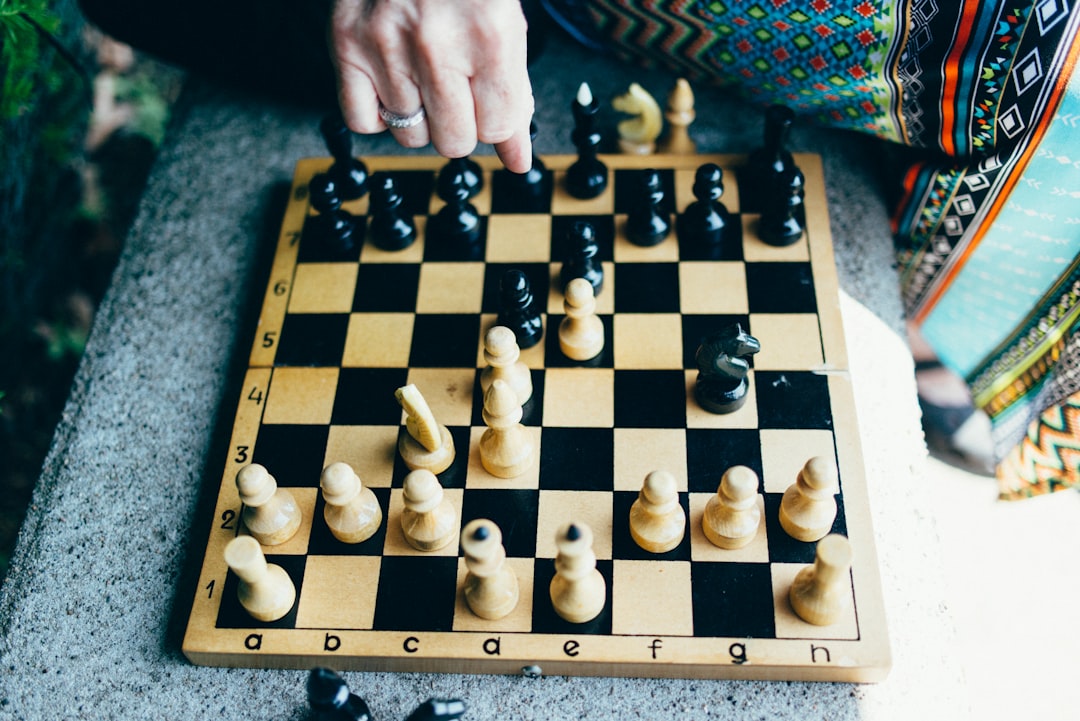
12. What is the difference between override
and final
?
override
: Shows that a function is meant to override a base class method.final
: Prevents further overriding or inheritance.
These add clarity and safety to your code.
13. How do templates help in generic programming?
Templates let you write a function or class to work with any data type. It’s like giving your code superpowers for reusability.
14. What’s the difference between std::map
and std::unordered_map
?
- std::map: Ordered, implemented using balanced trees.
- std::unordered_map: Faster average access, uses hash tables, unordered.
15. What are the most common pitfalls in multithreading?
Multithreading is powerful but tricky. Common issues:
- Deadlocks
- Race conditions
- Improper locking
Use mutexes, lock guards, and tools like std::thread
carefully!
Final Thoughts
These questions focus on core and advanced C++ concepts. Mastering them means you can solve practical problems with elegance — and impress interviewers!
Remember: understand the why, not just the how. And always show your passion for writing clean, efficient code.
Happy coding and good luck with that interview!
Leave a Reply